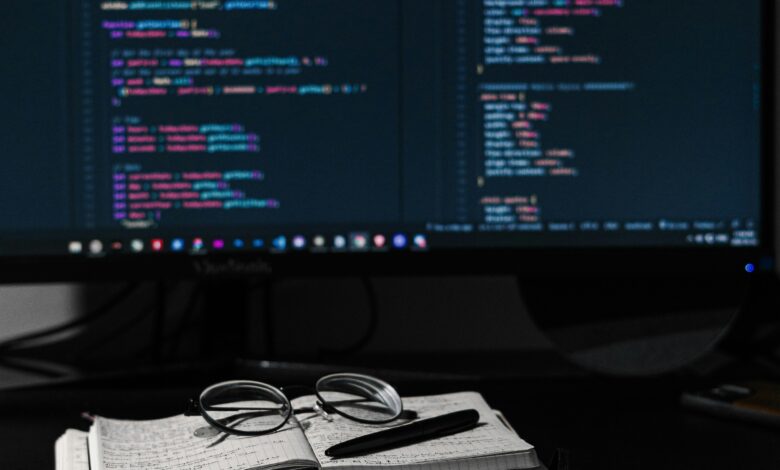
Selenium is a leading tool for web application testing, known for its flexibility and compatibility with various browsers. Its ability to automate nearly every web interaction makes it a popular choice among testers.
One of the key advantages of Selenium is its support for multiple programming languages, with Selenium Python being particularly favored for its simplicity, readability, and extensive libraries. This combination allows users to create effective, scalable, and maintainable automation frameworks. For beginners, writing basic test cases with Selenium Python can be straightforward, such as verifying user interactions or checking if a page load correctly.
As web applications become more complex, testing scenarios also increase in difficulty. AJAX-heavy websites, dynamic content, and cross-browser compatibility require advanced techniques beyond basic automation. Expert-level methods are essential to ensure tests remain effective and reliable.
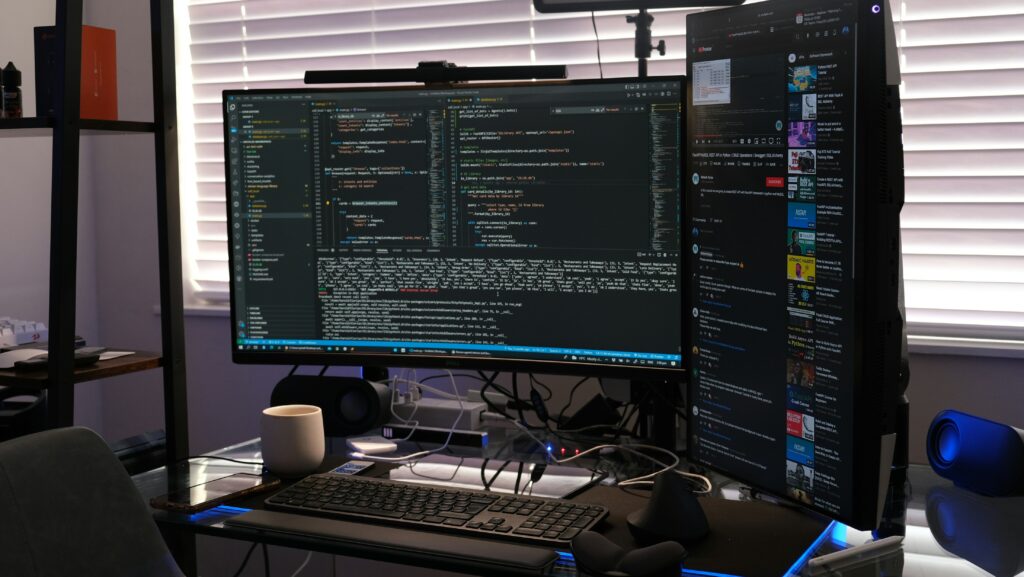
1. Optimizing WebDriver Setup
The central component of Selenium is the WebDriver, which serves as a conduit for your Python scripts and the web browser. Optimizing WebDriver management is the first step towards making your tests scalable and effective.
- Headless Browser Testing: Using an advanced technique called headless browser testing, you can run Selenium tests without a visible browser interface. While the browser is in headless mode, it runs in the background, carrying out all test actions and interactions without displaying the graphical user interface (GUI). Rendering the user interface (UI) can require a lot of resources, especially when working with large test suites or numerous test cases. This approach greatly reduces resource consumption.Ā
For scenarios where graphical resources are scarce, like in CI/CD pipelines, remote servers, or cloud-based testing platforms, headless browsers like Chrome and Firefox are perfect. In addition to saving system resources, headless testing enhances scalability and enables parallel test execution across several environments. This method helps shorten test cycles while preserving accuracy, which is especially useful for regression testing, where frequent test runs are required to guarantee application stability.
- Browser Profiles: A crucial tool for modifying the browser environment in complex Selenium testing scenarios is a browser profile. You can ensure that the browser behaves in a controlled and consistent way throughout test executions by pre-configuring particular settings and preferences by creating a browser profile. This can serve multiple purposes like configuring the browser to automatically turn off extensions that might interfere with test automation or preloading particular cookies to preserve user sessions across test cases.Ā
- Furthermore, security alerts like pop-up windows or certificate errors that might otherwise impede or slow down the test flow can be turned off via browser profiles. To avoid disruptions during testing, you can establish a custom profile that automatically accepts self-signed certificates in the staging environment. You can more closely mimic real-world user settings by using browser profiles.Ā
Parallel Testing: The time required to finish large test suites can be significantly reduced by using parallel testing, which runs multiple test cases simultaneously. Python frameworks such as pytest-xdist enable parallel execution of Selenium test cases across multiple CPU cores or even separate machines. It can be laborious and inefficient to run tests sequentially when dealing with hundreds or thousands of tests.
It is very beneficial in continuous integration (CI) environments where developers require prompt feedback to identify issues early in the development cycle. Enhancing development velocity and facilitating faster releases ensures faster validation of code changes and speeds up the entire testing process.
Furthermore, parallel testing can be scaled quickly, so as your test suite grows, you can add more processing power without sacrificing the speed of execution.
2. Handling Dynamic Web Elements
When it comes to automation testing, working with dynamic web elements that regularly change their ID classes or even locations is one of the most difficult tasks.
- XPath and CSS Selectors: In dynamic applications where elements may lack stable attributes like IDs or classes, mastering advanced XPath and CSS selectors is essential for effectively identifying and interacting with web elements.Ā
Elements that are deeply nested in the DOM or lack unique identifiers can be precisely located by testers thanks to CSS and XPath selectors. Instead of depending just on fixed attributes, advanced XPath techniquesālike relative XPathsāallow you to navigate the DOM hierarchy by referencing the structure or relationships between elements.
Relative XPaths for instance, can be used to target elements based on how they are positioned about other known elements like parents or siblings. This is especially helpful when elements are constantly changing as a result of dynamic content loading.
- Shadow DOM: More and more web apps are using Shadow DOM to encapsulate sections of the DOM tree as they develop. With Selenium you can navigate the Shadow DOM and find elements that are nested inside of it.Ā
- JavaScript Execution: You can use Selenium’s JavaScript execution capabilities to directly interact with dynamic elements in situations where traditional element locators are unable to work.
3. Efficient Use of Selenium Waits
It’s common for testing dynamic applications to involve handling asynchronous loading elements. This can result in erratic test failures if not managed appropriately.
To deal with this:
- Implicit vs. Explicit Waits: To ensure that web elements are available before your test interacts with them, explicit and implicit waits are crucial synchronization techniques in Selenium that help lower the risk of test failures brought on by timing issues. When Selenium tries to find any element on the page, implicit waits instruct it to wait for a predetermined period. If Selenium cannot find the element right away it will keep polling the DOM until it does or the time limit runs out.Ā
- Fluent Waits: These waits let you set a maximum timeout and polling intervals for expert-level handling so your tests won’t be delayed any longer than necessary.
4. Cross-Browser Testing
Web apps nowadays need to function flawlessly on a variety of browsers and hardware. Selenium WebDriver supports all of the popular browsers, although cross-browser testing can still present difficulties.
- LambdaTest Integration: You can run tests on multiple browser/OS combinations by integrating Selenium Python tests with cloud-based platforms such as LambdaTest. Additionally, LambdaTest allows for parallel testing in various browser environments which cuts down on testing time dramatically.Ā
- Capabilities Management: To achieve advanced automation, distinct browser capabilities must be defined. Layout bugs that might go undetected in desktop environments can be found for instance,e by testing in mobile viewports or setting custom resolution sizes.
5. Managing Test Data
Managing test data becomes essential as your test suite grows to keep your automation scripts flexible, scalable, and reusable. Your scripts may become stiff, prone to errors, and challenging to update or modify to accommodate new scenarios if test data is hardcoded directly into them. Your tests can become more dynamic and reusable in a variety of settings and use cases by externalizing test data.
- Data-Driven Testing: Test logic can be isolated from actual test data using the effective technique known as data-driven testing. You store data in external files like CSV, JSON, Excel, or even databases rather than directly embedding it in the test scripts for things like user inputs, URLs, or expected outputs.Ā
- To increase test coverage without writing duplicate code this enables you to run the same test case several times with various inputs. It is simple to integrate testing frameworks such as unittest or pytest with external data sources to retrieve and repeat over various data sets, guaranteeing that your test cases are flexible and adjustable. This approach minimizes maintenance work because you just need to update the external data files rather than changing the test scripts which increases the reusability of your tests.
- Environment-Specific Data: In more complex situations, environment-specific data management is crucial when your application is used in development, staging, and production, among other environments. Distinct setups such as alternative URLs, database connections, API keys, or credentials might be necessary for every environment. You can set up your tests to dynamically load the appropriate environment-specific data at runtime to make them more flexible.
6. Integrating with Continuous Integration (CI) Pipelines
- CI Tools: To preserve code quality, speed up development cycles, and guarantee that applications stay stable as new changes are implemented. Large-scale projects must automate the execution of Selenium Python tests in a Continuous Integration (CI) environment.Ā
Software development lifecycles can incorporate automated tests with the help of continuous integration (CI) tools like Travis CI, GitLab CI, Jenkins CI, and CircleCI. Through the automatic triggering of tests upon code commit push or merge, Selenium tests integrated into continuous integration pipelines enable teams to continuously confirm that new features or updates do not introduce new bugs or break existing functionality.
- Test Reporting: Test reporting is an essential component of continuous integration pipelines. A clear and comprehensive test report that aids developers and testers in quickly comprehending test results and troubleshooting issues is essential for optimizing automated testing.Ā
Many reporting formats are supported by continuous integration (CI) tools, with JUnit XML being one of the most popular. The pipeline can provide a thorough summary of test results, including passed, failed, and skipped tests, because this format easily integrates with continuous integration (CI) tools).
Automation Testing with LambdaTest: A Guide to Seamless Cross-Browser Testing
A cloud-based cross-browser testing tool called LambdaTest was developed to facilitate and ease the testing of websites on a variety of devices. With LambdaTest’s extensive testing features, developers can make sure that their websites are optimized and provide a consistent user experience for every user regardless of the browser or device they’re using.
LambdaTest is an AI-driven platform for test orchestration and execution that allows developers and QA specialists to run automated tests on over 3000 environments, including real-world scenarios.
Making sure your web application works properly across different browsers, devices, and operating systems is essential for providing a consistent user experience. However, it can be expensive and time-consuming to set up and maintain several testing environments. This is where LambdaTest comes i. It’s a cloud-based cross-browser testing platform that makes testing easier by enabling teams to run automated tests across thousands of OS and browser combinations without requiring physical infrastructure.
You can run your Selenium Python tests on multiple browsers simultaneously with LambdaTest’s seamless integration with automation tools like Selenium. This can greatly accelerate your testing process. Weāll look at how to use LambdaTest below to increase the effectiveness and coverage of your automation testing.
- Parallel Testing: Large test suite execution times can be greatly decreased by using LambdaTest to run multiple tests in parallel. Fast feedback is crucial in Continuous Integration (CI) pipelines to make sure that new code changes don’t affect already-existing functionality, so this capability is extremely helpful in these situations.
- No Infrastructure Management: Physical infrastructure management and maintenance are not a concern with LambdaTest because it is a cloud-based platform. It is simpler and faster to set up your test environment when you can access a large range of browsers and devices directly through the cloud.
Conclusion:
Gaining proficiency in Python and Selenium opens up a world of opportunities for automating sophisticated web applications. Your test suite resilience can be greatly increased as you move beyond basic automation by utilizing sophisticated strategies like dynamic element handling, optimized waits, and continuous integration. Further improving the scalability and maintainability of your tests is the use of tools such as LambdaTest for cross-browser testing and appropriate data management. The foundation of dependable, scalable automation, according to seasoned testers, is comprehensive testing and continual optimization. Continue testing and improving your strategies to guarantee consistent user experiences on all platforms.
With LambdaTest, teams can easily scale their Selenium Python tests across multiple environments by providing an all-inclusive solution for automating cross-browser testing. You can guarantee that your web applications are tested thoroughly, quickly, and effectively without having to worry about managing complicated infrastructure by incorporating LambdaTest into your development workflow. LambdaTest gives development teams the tools they need to produce high-quality software quickly and confidently with features like parallel testing, continuous integration, and comprehensive reporting.